In a previous article we looked at the scroll:bit, this is an add on from Pimoroni which contains a lot of LEDs, in fact there are 119 in total in a 17 x 7 matrix – its controlled by a IS31FL3731 LED matrix driver chip.
This is what the scroll:bit looks like fitted to a bpi:bit
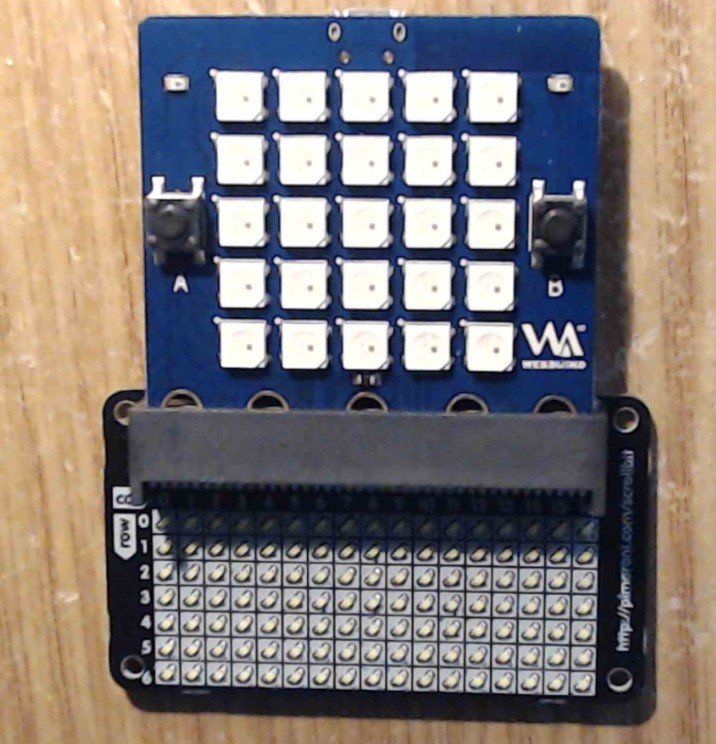
bpi:bit and scroll:bit
Parts List
The scroll:bit costs about $18.99 from the US.
Here are a couple of links to the products
Part | Link |
---|---|
Banana PI Bit board | Banana PI Bit board with ESP32 |
scroll:bit | Pimoroni Scroll:bit |
Code
You need to to install the Adafruit_GFX and Adafruit_IS31FL3731 libraries, you can add these using the library manager.
The key thing is that the Featherwing version is the same size as the scroll:bit. So in the code you need to use
Adafruit_IS31FL3731_Wing ledmatrix = Adafruit_IS31FL3731_Wing();
These 2 examples work fairly well
[codesyntax lang=”cpp”]
#include <Wire.h> #include <Adafruit_GFX.h> #include <Adafruit_IS31FL3731.h> // If you're using the full breakout... //Adafruit_IS31FL3731 ledmatrix = Adafruit_IS31FL3731(); // If you're using the FeatherWing version Adafruit_IS31FL3731_Wing ledmatrix = Adafruit_IS31FL3731_Wing(); // The lookup table to make the brightness changes be more visible uint8_t sweep[] = {1, 2, 3, 4, 6, 8, 10, 15, 20, 30, 40, 60, 60, 40, 30, 20, 15, 10, 8, 6, 4, 3, 2, 1}; void setup() { Serial.begin(9600); Serial.println("ISSI swirl test"); if (! ledmatrix.begin()) { Serial.println("IS31 not found"); while (1); } Serial.println("IS31 found!"); } void loop() { // animate over all the pixels, and set the brightness from the sweep table for (uint8_t incr = 0; incr < 24; incr++) for (uint8_t x = 0; x < 16; x++) for (uint8_t y = 0; y < 9; y++) ledmatrix.drawPixel(x, y, sweep[(x+y+incr)%24]); delay(20); }
[/codesyntax]
and now for the second example
[codesyntax lang=”cpp”]
#include <Wire.h> #include <Adafruit_GFX.h> #include <Adafruit_IS31FL3731.h> // If you're using the full breakout... //Adafruit_IS31FL3731 matrix = Adafruit_IS31FL3731(17,7); // If you're using the FeatherWing version Adafruit_IS31FL3731_Wing matrix = Adafruit_IS31FL3731_Wing(); static const uint8_t PROGMEM smile_bmp[] = { B00111100, B01000010, B10100101, B10000001, B10100101, B10011001, B01000010, B00111100 }, neutral_bmp[] = { B00111100, B01000010, B10100101, B10000001, B10111101, B10000001, B01000010, B00111100 }, frown_bmp[] = { B00111100, B01000010, B10100101, B10000001, B10011001, B10100101, B01000010, B00111100 }; void setup() { Serial.begin(9600); Serial.println("ISSI manual animation test"); if (! matrix.begin()) { Serial.println("IS31 not found"); while (1); } Serial.println("IS31 Found!"); } void loop() { matrix.setRotation(0); matrix.clear(); matrix.drawBitmap(3, 0, smile_bmp, 8, 8, 255); delay(500); matrix.clear(); matrix.drawBitmap(3, 0, neutral_bmp, 8, 8, 64); delay(500); matrix.clear(); matrix.drawBitmap(3, 0, frown_bmp, 8, 8, 32); delay(500); matrix.clear(); matrix.drawPixel(0, 0, 255); delay(500); matrix.clear(); matrix.drawLine(0,0, matrix.width()-1, matrix.height()-1, 127); delay(500); matrix.clear(); matrix.drawRect(0,0, matrix.width(), matrix.height(), 255); matrix.fillRect(2,2, matrix.width()-4, matrix.height()-4, 20); delay(500); matrix.clear(); matrix.drawCircle(8,4, 4, 64); matrix.drawCircle(8,4, 2, 32); delay(500); matrix.setTextSize(1); matrix.setTextWrap(false); // we dont want text to wrap so it scrolls nicely matrix.setTextColor(100); for (int8_t x=0; x>=-32; x--) { matrix.clear(); matrix.setCursor(x,0); matrix.print("Hello"); delay(100); } matrix.setTextSize(2); matrix.setTextWrap(false); // we dont want text to wrap so it scrolls nicely matrix.setTextColor(32); matrix.setRotation(1); for (int8_t x=7; x>=-64; x--) { matrix.clear(); matrix.setCursor(x,0); matrix.print("World"); delay(100); } }
[/codesyntax]
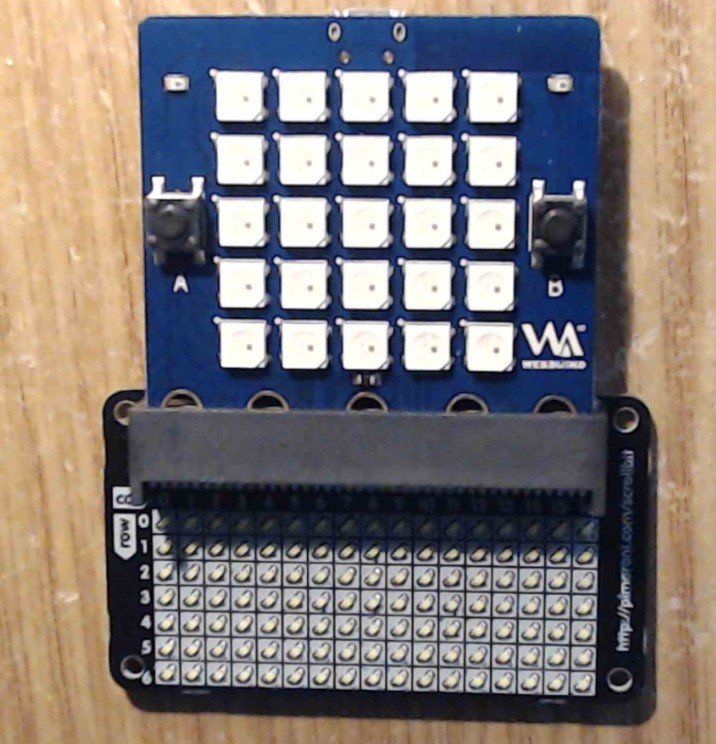
bpi:bit and scroll:bit