The Si7021 I2C Humidity and Temperature Sensor is a monolithic CMOS IC integrating humidity and temperature sensor elements, an analog-to-digital converter, signal processing, calibration data, and an I2C Interface. The Si7021 offers an accurate, low-power, factory-calibrated digital solution ideal for measuring humidity, dew-point, and temperature, in applications ranging from HVAC/R and asset tracking to industrial and consumer platforms.
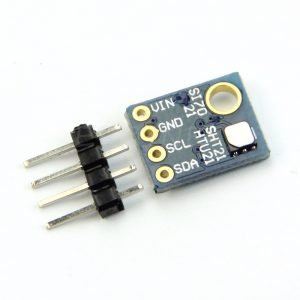
Si7021
Features
Precision Relative Humidity Sensor ± 3% RH (max), 0–80% RH
High Accuracy Temperature Sensor ±0.4 °C (max), –10 to 85 °C
0 to 100% RH operating range
Up to –40 to +125 °C operating range
Wide operating voltage – (1.9 to 3.6 V)
Low Power Consumption – 150 μA active current
Parts Required
Here are the parts I used
Connection
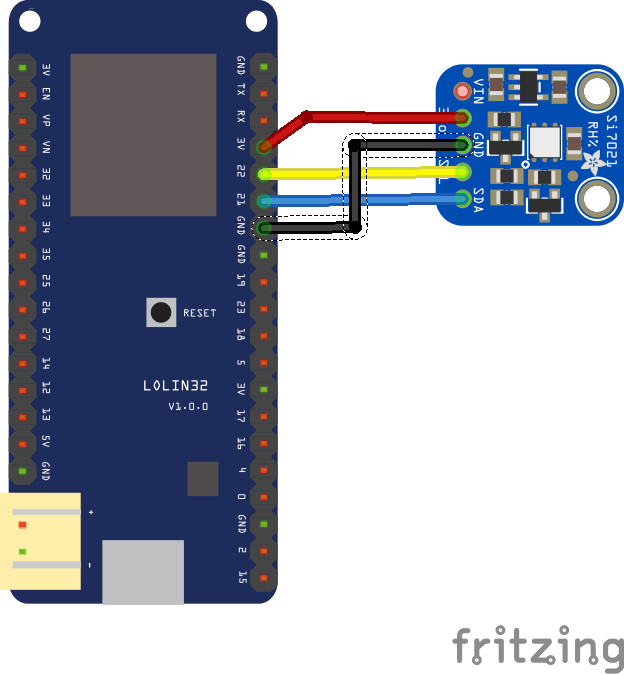
esp32 and si7021
Code
No 3rd party libraries required
#include <Wire.h> // SI7021 I2C address is 0x40(64) #define si7021Addr 0x40 void setup() { Wire.begin(); Serial.begin(9600); Wire.beginTransmission(si7021Addr); Wire.endTransmission(); delay(300); } void loop() { unsigned int data[2]; Wire.beginTransmission(si7021Addr); //Send humidity measurement command Wire.write(0xF5); Wire.endTransmission(); delay(500); // Request 2 bytes of data Wire.requestFrom(si7021Addr, 2); // Read 2 bytes of data to get humidity if(Wire.available() == 2) { data[0] = Wire.read(); data[1] = Wire.read(); } // Convert the data float humidity = ((data[0] * 256.0) + data[1]); humidity = ((125 * humidity) / 65536.0) - 6; Wire.beginTransmission(si7021Addr); // Send temperature measurement command Wire.write(0xF3); Wire.endTransmission(); delay(500); // Request 2 bytes of data Wire.requestFrom(si7021Addr, 2); // Read 2 bytes of data for temperature if(Wire.available() == 2) { data[0] = Wire.read(); data[1] = Wire.read(); } // Convert the data float temp = ((data[0] * 256.0) + data[1]); float celsTemp = ((175.72 * temp) / 65536.0) - 46.85; float fahrTemp = celsTemp * 1.8 + 32; // Output data to serial monitor Serial.print("Humidity : "); Serial.print(humidity); Serial.println(" % RH"); Serial.print("Celsius : "); Serial.print(celsTemp); Serial.println(" C"); Serial.print("Fahrenheit : "); Serial.print(fahrTemp); Serial.println(" F"); delay(1000); }
Testing
Open the serial monitor and you should see something like this
Humidity : 44.89 % RH
Celsius : 21.07 C
Fahrenheit : 69.93 F
Humidity : 45.25 % RH
Celsius : 22.14 C
Fahrenheit : 71.86 F
Humidity : 47.32 % RH
Celsius : 26.53 C
Fahrenheit : 79.76 F
Humidity : 49.03 % RH
Celsius : 28.33 C
Fahrenheit : 83.00 F
Humidity : 50.74 % RH
Celsius : 29.26 C
Fahrenheit : 84.66 F
Link