In this example we connect a GY-21P sensor to an ESP32 and then we will display the readings on a webpage
Lets recap the GY-21P module which spoke about in a previous article
The GY-21P is an interesting module in that it combines a BMP280 sensor and an SI7021 sensor. The on-board BMP280+SI7021 sensor measures atmospheric pressure from 30kPa to 110kPa as well as relative humidity and temperature.
BMP280
Pressure range: 300-1100 hPa (9000 meters above sea level at -500m)
Relative accuracy (at 950 – 1050 hPa at 25 ° C): ± 0.12 hPa, equiv. to ± 1 m
Absolute accuracy (at (950 – 1050 hPa, 0 – +40 ° C): ± 0.12 hPa, equiv. To ± 1 m
Mains voltage: 1.8V – 3.6V
Power consumption: 2.7µA at 1Hz readout rate
Temperature range: -40 to + 85 ° C
SI7021
HVAC/R
Thermostats/humidistats
Respiratory therapy
White goods
Indoor weather stations
Micro-environments/data centers
Automotive climate control and defogging
Asset and goods tracking
Mobile phones and tablets
Size: 1.3*1cm/0.51*0.39″
Features:
Operation Voltage: 3.3V
I2C & SPI Communications Interface
Temp Range: -40C to 85C
Humidity Range: 0 – 100% RH, =-3% from 20-80%
Pressure Range: 30,000Pa to 110,000Pa, relative accuracy of 12Pa, absolute accuracy of 100Pa
Altitude Range: 0 to 30,000 ft (9.2 km), relative accuracy of 3.3 ft (1 m) at sea level, 6.6 (2 m) at 30,000 ft.
Parts List
Schematics/Layout
Connect the sensor to the ESP32
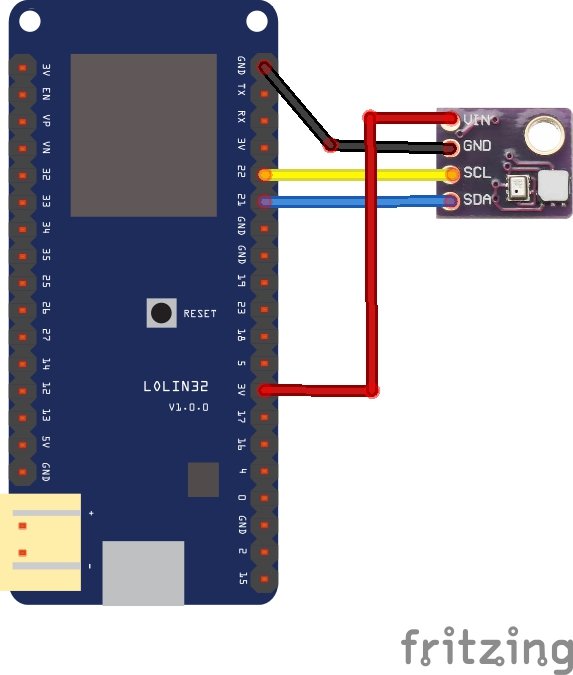
esp32 and GY21P
Code
I use a variety of Adafruit libraries, took the default examples and made the following out of them
https://github.com/adafruit/Adafruit_Sensor
https://github.com/adafruit/Adafruit_BMP280_Library
https://github.com/adafruit/Adafruit_Si7021
I got the sea level pressure value from this link
#include <WiFi.h> #include <Wire.h> #include <Adafruit_Sensor.h> #include <Adafruit_BMP280.h> #include "Adafruit_Si7021.h" const char* ssid = "yourname"; const char* password = "yourpasssword"; Adafruit_BMP280 bmp; // I2C Adafruit_Si7021 sensor = Adafruit_Si7021(); WiFiServer server(80); void setup() { Serial.begin(115200); pinMode(5, OUTPUT); // set the LED pin mode if (!bmp.begin()) { Serial.println("Could not find a valid BMP280 sensor, check wiring!"); while (1); } if (!sensor.begin()) { Serial.println("Did not find Si7021 sensor!"); while (true); } delay(10); // We start by connecting to a WiFi network Serial.println(); Serial.println(); Serial.print("Connecting to "); Serial.println(ssid); WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { delay(500); Serial.print("."); } Serial.println(""); Serial.println("WiFi connected."); Serial.println("IP address: "); Serial.println(WiFi.localIP()); server.begin(); } int value = 0; void loop(){ WiFiClient client = server.available(); // listen for incoming clients if (client) { // if you get a client, Serial.println("New Client."); // print a message out the serial port String currentLine = ""; // make a String to hold incoming data from the client while (client.connected()) { // loop while the client's connected if (client.available()) { // if there's bytes to read from the client, char c = client.read(); // read a byte, then Serial.write(c); // print it out the serial monitor if (c == '\n') { // if the byte is a newline character // if the current line is blank, you got two newline characters in a row. // that's the end of the client HTTP request, so send a response: if (currentLine.length() == 0) { client.println("HTTP/1.1 200 OK"); client.println("Content-Type: text/html"); client.println("Connnection: close"); client.println(); client.println("<!DOCTYPE HTML>"); client.println("<html>"); client.println("<meta http-equiv=\"refresh\" content=\"5\">"); client.println("<br />"); //bmp280 part client.println("<h3>BMP280 readings</h3>"); client.print("Pressure (Pa): "); client.println((float)bmp.readPressure(), 1); client.println("<br />"); client.print("Temperature (C): "); client.println((float)bmp.readTemperature(), 1); client.println("<br />"); client.print("Altitude (m): "); client.println((float)bmp.readAltitude(1024), 1); // this should be adjusted to your local forcase client.println("<br />"); //SI7021 part client.println("<h3>SI7021 readings</h3>"); client.print("Humidity (%): "); client.println((float)sensor.readHumidity(), 1); client.println("<br />"); client.print("Temperature (C): "); client.println((float)sensor.readTemperature(), 1); client.println("<br />"); client.println("</html>"); break; // break out of the while loop: break; } else { // if you got a newline, then clear currentLine: currentLine = ""; } } else if (c != '\r') { // if you got anything else but a carriage return character, currentLine += c; // add it to the end of the currentLine } } } // close the connection: client.stop(); Serial.println("Client Disconnected."); } }
Output
OPen the serial monitor and take a note of the IP address
Open your favourite web browser and type in the IP address from above, you should see something like this
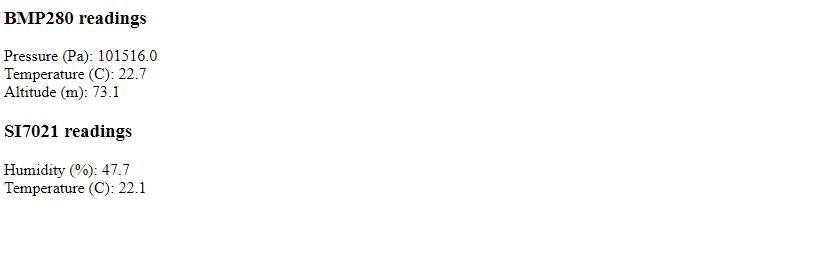
gy-21p output
Links