The MMA7455 accelerometer is a sensor that can measure acceleration in three axes. This sensor is commonly available as a breakout board that you can connect to your Arduino. It requires VCC, GND , SCA and SCL to be connected.
The MMA7455L is a Digital Output (I2C/SPI), low power, low profile capacitive micromachined accelerometer featuring signal conditioning, a low pass filter, temperature compensation, self-test, configurable to detect 0g through interrupt pins (INT1 or INT2), and pulse detect for quick motion detection.
0g offset and sensitivity are factory set and require no external devices. The 0g offset can be customer calibrated using assigned 0g registers and g-Select which allows for command selection for 3 acceleration ranges (2g/4g/8g).
The MMA7455L includes a Standby Mode that makes it ideal for handheld battery powered electronics.
Features
• Digital Output (I2C/SPI)
• 3mm x 5mm x 1mm LGA-14 Package
• Self-Test for Z-Axis
• Low Voltage Operation: 2.4 V – 3.6 V
• User Assigned Registers for Offset Calibration
• Programmable Threshold Interrupt Output
• Level Detection for Motion Recognition (Shock, Vibration, Freefall)
• Pulse Detection for Single or Double Pulse Recognition
• Sensitivity (64 LSB/g @ 2g and @ 8g in 10-Bit Mode)
• Selectable Sensitivity (±2g, ±4g, ±8g) for 8-bit Mode
• Robust Design, High Shocks Survivability (5,000g)
Here is a picture of the sensor breakout.
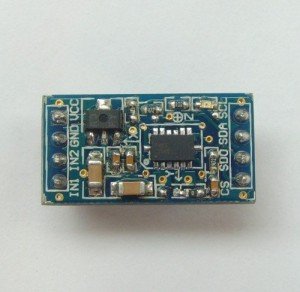
MMA7455 Accelerometer Sensor Module
Here is how to wire the MMA7455 accelerometer to your LOLIN32
Parts Required
Here are the parts I used
The sensor you can pick up in the $6 price range – you can connect to the sensor using a standard header the classic dupont style jumper wire.
Schematic/Layout
Its an I2c device, so connect to the I2C pins of your ESP32 module
Code
You will need a copy of the https://github.com/eklex/MMA_7455 to make your life easier. Download and copy to your Arduino -> Libraries folder
This is one of the test examples
#include <SPI.h> #include <Wire.h> #include <MMA_7455.h> /* Case 1: Accelerometer on the I2C bus (most common) */ MMA_7455 accel = MMA_7455(i2c_protocol); /* Case 2: Accelerometer on the SPI bus with CS on pin 2 */ // MMA_7455 accel = MMA_7455(spi_protocol, A2); int16_t x10, y10, z10; float xg, yg, zg; void setup() { /* Set serial baud rate */ Serial.begin(9600); /* Start accelerometer */ accel.begin(); /* Set accelerometer sensibility */ accel.setSensitivity(2); /* Verify sensibility - optional */ if(accel.getSensitivity() != 2) Serial.println("Sensitivity failure"); /* Set accelerometer mode */ accel.setMode(measure); /* Verify accelerometer mode - optional */ if(accel.getMode() != measure) Serial.println("Set mode failure"); /* Set axis offsets */ /* Note: the offset is hardware specific * and defined thanks to the auto-calibration example. */ accel.setAxisOffset(0, 0, 0); } void loop() { /* Get 10-bit axis raw values */ x10 = accel.readAxis10('x'); y10 = accel.readAxis10('y'); z10 = accel.readAxis10('z'); /* Get 10-bit axis values in g */ xg = accel.readAxis10g('x'); yg = accel.readAxis10g('y'); zg = accel.readAxis10g('z'); /* Display current axis values */ Serial.print("X: "); Serial.print(x10, DEC); Serial.print("\tY: "); Serial.print(y10, DEC); Serial.print("\tZ: "); Serial.print(z10, DEC); Serial.print("\tXg: "); Serial.print(xg, DEC); Serial.print("\tYg: "); Serial.print(yg, DEC); Serial.print("\tZg: "); Serial.print(zg, DEC); Serial.println(); delay(500); }
Output
Open the serial monitor window
X: -65 Y: -15 Z: 44 Xg: -0.2539062500 Yg: -0.0585937500 Zg: 0.1718750000
X: -65 Y: -15 Z: 44 Xg: -0.2539062500 Yg: -0.0585937500 Zg: 0.1718750000
X: -65 Y: -15 Z: 44 Xg: -0.2539062500 Yg: -0.0585937500 Zg: 0.1757812500
X: -62 Y: -46 Z: 41 Xg: -0.2343750000 Yg: -0.1718750000 Zg: 0.1562500000
X: -56 Y: 71 Z: 90 Xg: -0.2187500000 Yg: 0.2773437500 Zg: 0.3515625000
X: 20 Y: 70 Z: -85 Xg: 0.0781250000 Yg: 0.2734375000 Zg: -0.3320312500
X: -120 Y: 26 Z: -7 Xg: -0.4687500000 Yg: 0.0937500000 Zg: -0.0273437500
X: 9 Y: -62 Z: 33 Xg: 0.0468750000 Yg: -0.2460937500 Zg: 0.1015625000
X: 49 Y: -34 Z: 9 Xg: 0.1914062500 Yg: -0.1328125000 Zg: 0.0351562500
X: -15 Y: -92 Z: 32 Xg: -0.0585937500 Yg: -0.0781250000 Zg: 0.0468750000
X: 26 Y: -18 Z: -26 Xg: 0.1835937500 Yg: -0.1054687500 Zg: -0.1015625000
X: 40 Y: -18 Z: -26 Xg: 0.1562500000 Yg: -0.0703125000 Zg: -0.1015625000
Links
https://www.nxp.com/docs/en/data-sheet/MMA7455L.pdf