The ADXL345 is well suited for mobile device applications. It measures the static acceleration of gravity in tilt-sensing applications, as well as dynamic acceleration resulting from motion or shock. Its high resolution (4 mg/LSB) enables measurement of inclination changes less than 1.0°.
Several special sensing functions are provided. Activity and inactivity sensing detect the presence or lack of motion and if the acceleration on any axis exceeds a user-set level. Tap sensing detects single and double taps. Free-fall sensing detects if the device is falling. These functions can be mapped to one of two interrupt output pins. An integrated, patent pending 32-level first in, first out (FIFO) buffer can be used to store data to minimize host processor intervention.
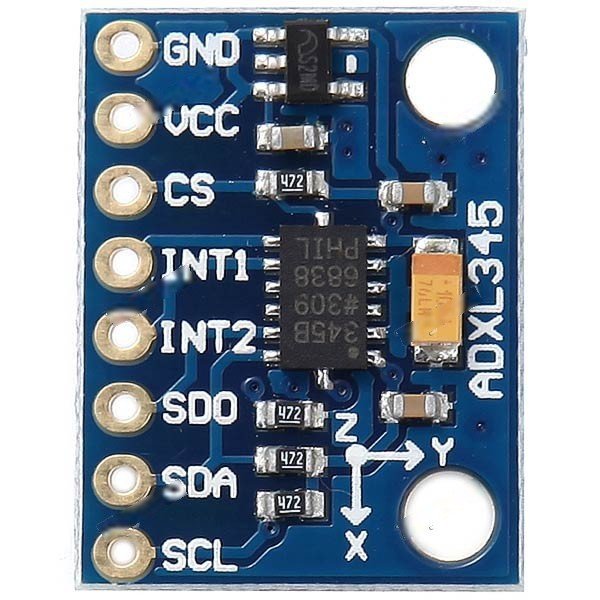
adxl345 module
Parts Required
Here are the parts I used
Connection and layout
I used the following connection from the module above to my Wemos Mini
LOLIN32 Connection | Module Connection |
3v3 | VCC |
Gnd | Gnd |
SDA 21 | SDA |
SCL 22 | SCL |
Here is a layout for reference
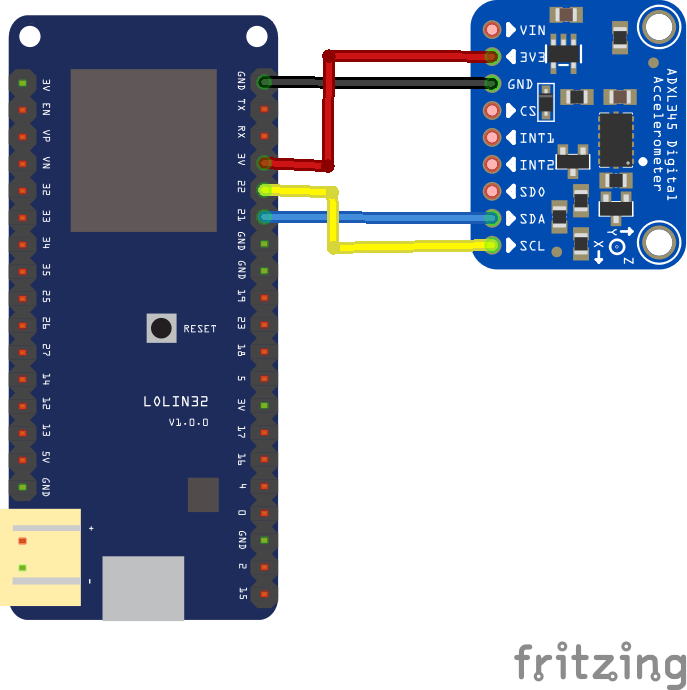
lolin32 and adxl345
Code
This needs the Adafruit library – https://github.com/adafruit/Adafruit_ADXL345
This is just the sensor test code from the library above
#include <Wire.h> #include <Adafruit_Sensor.h> #include <Adafruit_ADXL345_U.h> /* Assign a unique ID to this sensor at the same time */ Adafruit_ADXL345_Unified accel = Adafruit_ADXL345_Unified(12345); void displaySensorDetails(void) { sensor_t sensor; accel.getSensor(&sensor); Serial.println("------------------------------------"); Serial.print ("Sensor: "); Serial.println(sensor.name); Serial.print ("Driver Ver: "); Serial.println(sensor.version); Serial.print ("Unique ID: "); Serial.println(sensor.sensor_id); Serial.print ("Max Value: "); Serial.print(sensor.max_value); Serial.println(" m/s^2"); Serial.print ("Min Value: "); Serial.print(sensor.min_value); Serial.println(" m/s^2"); Serial.print ("Resolution: "); Serial.print(sensor.resolution); Serial.println(" m/s^2"); Serial.println("------------------------------------"); Serial.println(""); delay(500); } void displayDataRate(void) { Serial.print ("Data Rate: "); switch(accel.getDataRate()) { case ADXL345_DATARATE_3200_HZ: Serial.print ("3200 "); break; case ADXL345_DATARATE_1600_HZ: Serial.print ("1600 "); break; case ADXL345_DATARATE_800_HZ: Serial.print ("800 "); break; case ADXL345_DATARATE_400_HZ: Serial.print ("400 "); break; case ADXL345_DATARATE_200_HZ: Serial.print ("200 "); break; case ADXL345_DATARATE_100_HZ: Serial.print ("100 "); break; case ADXL345_DATARATE_50_HZ: Serial.print ("50 "); break; case ADXL345_DATARATE_25_HZ: Serial.print ("25 "); break; case ADXL345_DATARATE_12_5_HZ: Serial.print ("12.5 "); break; case ADXL345_DATARATE_6_25HZ: Serial.print ("6.25 "); break; case ADXL345_DATARATE_3_13_HZ: Serial.print ("3.13 "); break; case ADXL345_DATARATE_1_56_HZ: Serial.print ("1.56 "); break; case ADXL345_DATARATE_0_78_HZ: Serial.print ("0.78 "); break; case ADXL345_DATARATE_0_39_HZ: Serial.print ("0.39 "); break; case ADXL345_DATARATE_0_20_HZ: Serial.print ("0.20 "); break; case ADXL345_DATARATE_0_10_HZ: Serial.print ("0.10 "); break; default: Serial.print ("???? "); break; } Serial.println(" Hz"); } void displayRange(void) { Serial.print ("Range: +/- "); switch(accel.getRange()) { case ADXL345_RANGE_16_G: Serial.print ("16 "); break; case ADXL345_RANGE_8_G: Serial.print ("8 "); break; case ADXL345_RANGE_4_G: Serial.print ("4 "); break; case ADXL345_RANGE_2_G: Serial.print ("2 "); break; default: Serial.print ("?? "); break; } Serial.println(" g"); } void setup(void) { Serial.begin(9600); Serial.println("Accelerometer Test"); Serial.println(""); /* Initialise the sensor */ if(!accel.begin()) { /* There was a problem detecting the ADXL345 ... check your connections */ Serial.println("Ooops, no ADXL345 detected ... Check your wiring!"); while(1); } /* Set the range to whatever is appropriate for your project */ accel.setRange(ADXL345_RANGE_16_G); // displaySetRange(ADXL345_RANGE_8_G); // displaySetRange(ADXL345_RANGE_4_G); // displaySetRange(ADXL345_RANGE_2_G); /* Display some basic information on this sensor */ displaySensorDetails(); /* Display additional settings (outside the scope of sensor_t) */ displayDataRate(); displayRange(); Serial.println(""); } void loop(void) { /* Get a new sensor event */ sensors_event_t event; accel.getEvent(&event); /* Display the results (acceleration is measured in m/s^2) */ Serial.print("X: "); Serial.print(event.acceleration.x); Serial.print(" "); Serial.print("Y: "); Serial.print(event.acceleration.y); Serial.print(" "); Serial.print("Z: "); Serial.print(event.acceleration.z); Serial.print(" ");Serial.println("m/s^2 "); delay(500); }
Output
Open the serial monitor and you should get something like this
X: 4.55 Y: -0.90 Z: -6.67 m/s^2
X: -9.30 Y: 3.96 Z: -4.55 m/s^2
X: -10.43 Y: 2.47 Z: 5.92 m/s^2
X: -1.69 Y: 2.04 Z: -9.96 m/s^2
X: -2.59 Y: 7.96 Z: -4.20 m/s^2
X: -14.20 Y: 6.71 Z: -2.16 m/s^2
X: 3.30 Y: -2.90 Z: -8.04 m/s^2
X: 4.43 Y: -0.16 Z: -8.47 m/s^2
X: 1.45 Y: -2.63 Z: 15.38 m/s^2